版本:V6.0.0
背景
本文将简述ECharts
如何实现标签自动旋转特定角度以解决柱状图或折线图轴线标签重叠的问题。
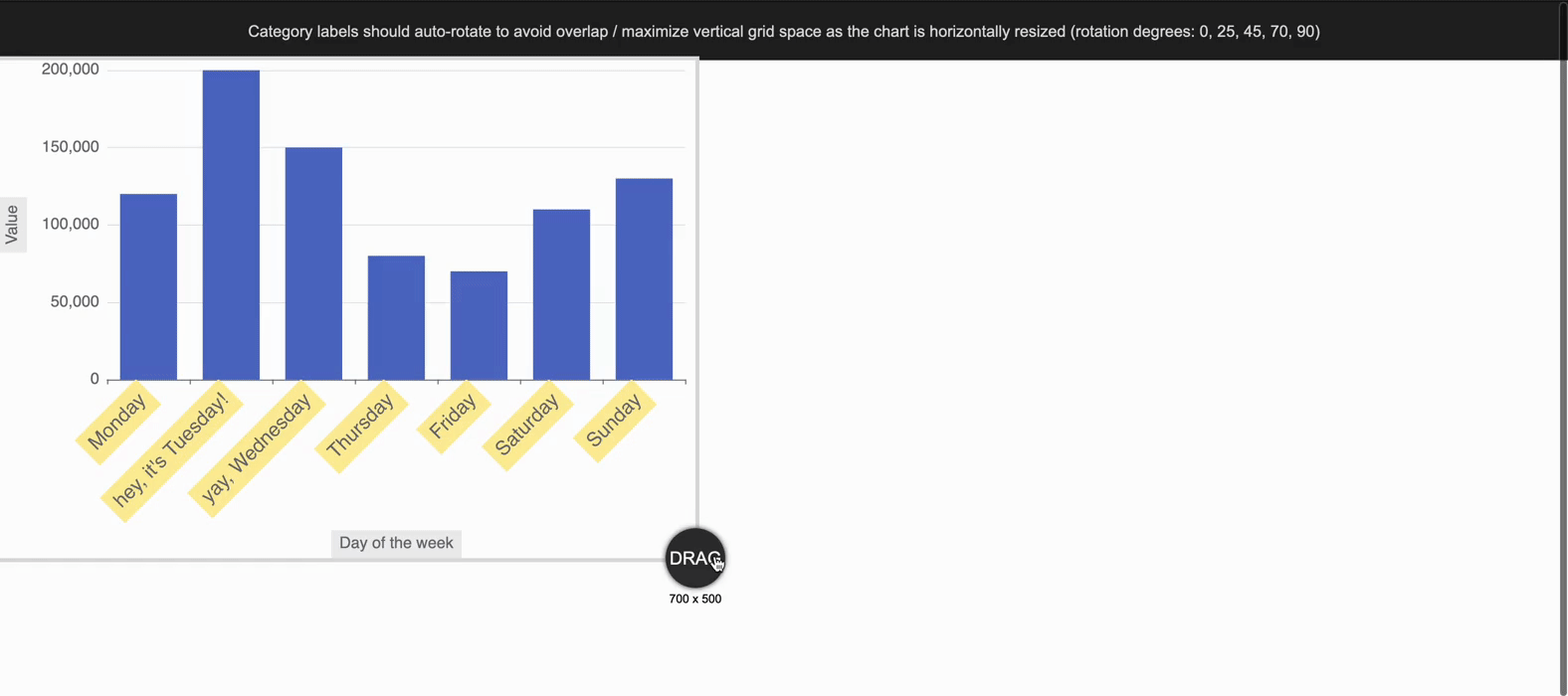
结论
解决问题的流程如下:
- 首先定义了几个候选的旋转角度
[0,25,45,70,90]
。
- 获取最长的
label
并根据最长的label
的 size
计算每一个候选的旋转角度下的size
(详情见:https://codepen.io/agurtovoy/pen/WNPyqWx)
- 其中
width
下图中的横向绿线部分即和轴交叉的部分。
- 其中
height
为下图中红框高度。
- 将步骤2的
size
和图表中的间隔做比对,横向图width
做比对纵向图height
做比对。看最大label
的交叉长度需要几个单位间隔的宽度来装。这个值即为label
的间隔个数。
- 计算所有的候选角度所需要的间隔数,选择所需间隔最少且其中角度最小作为自适应的结果。
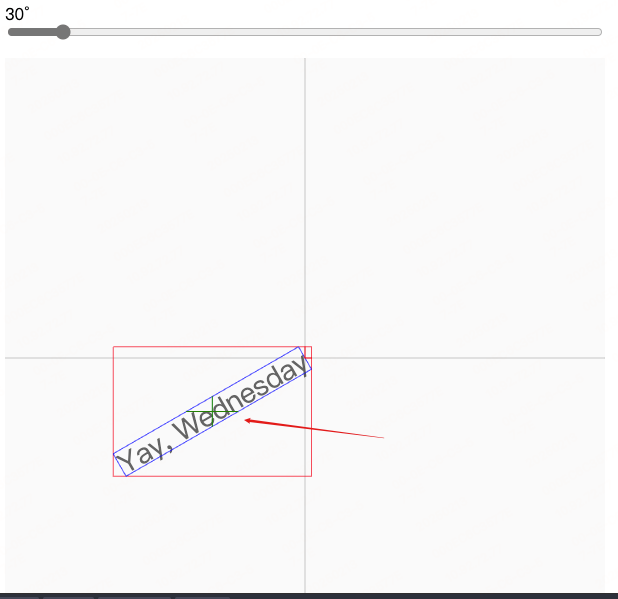
核心逻辑
生成标签旋转的候选布局
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| getCandidateLayouts(axis: Axis) { const unitSize = calculateUnitDimensions(axis); const maxLabelSize = calculateMaxLabelDimensions(axis); const isHorizontal = isHorizontalAxis(axis);
const labelModel = axis.getLabelModel(); const labelRotations = normalizeLabelRotations(getLabelRotations(labelModel), isHorizontal); const labelMinDistance = labelModel.get('minDistance') ?? 0;
const candidateLayouts = []; for (const rotation of labelRotations) { const { axesIntersection, bounds, offset } = rotateLabel(maxLabelSize, rotation); const labelSize = isHorizontal ? { width: axesIntersection.width, height: bounds.height - offset.y } : { width: bounds.width - offset.x, height: axesIntersection.height }; const interval = calculateLabelInterval(unitSize, labelSize, labelMinDistance); candidateLayouts.push({ labelSize, interval, rotation }); } return candidateLayouts; }
|
旋转标签
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
|
function rotateLabel({ width, height }: Size, rotation: number) { const rad = rotation * RADIAN; const sin = Math.abs(Math.sin(rad)); const cos = Math.abs(Math.cos(rad));
const axesIntersection = { width: Math.min(width / cos, height / sin), height: Math.min(height / cos, width / sin) };
const bounds = { width: width * cos + height * sin, height: width * sin + height * cos };
const asbRotation = Math.abs(rotation); const bboxOffset = asbRotation === 0 || asbRotation === 180 ? 0 : height / 2; const offset = { x: bboxOffset * sin, y: bboxOffset * cos };
return { axesIntersection, bounds, offset }; }
|
计算间隔
1 2 3 4 5 6 7 8 9 10 11 12 13
|
function calculateLabelInterval(unitSize: Size, maxLabelSize: Size, minDistance: number) { let dw = (maxLabelSize.width + minDistance) / unitSize.width; let dh = (maxLabelSize.height + minDistance) / unitSize.height; isNaN(dw) && (dw = Infinity); isNaN(dh) && (dh = Infinity);
return Math.max(0, Math.floor(Math.min(dw, dh))); }
|
选择布局
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| function chooseAutoLayout(candidateLayouts: InnerAutoLayoutCachedVal[]): InnerAutoLayoutCachedVal { let autoLayout = { interval: Infinity, rotation: 0 }; for (const layout of candidateLayouts) { if (layout.interval < autoLayout.interval || layout.interval > 0 && layout.interval === autoLayout.interval) { autoLayout = layout; } }
return autoLayout; }
|
参考 & 引用
https://github.com/apache/echarts/pull/19348
https://codepen.io/agurtovoy/pen/WNPyqWx
https://math.stackexchange.com/questions/1449352/intersection-between-a-side-of-rotated-rectangle-and-axis
https://i.sstatic.net/u7XIE.png